There are too many Javascript frameworks!
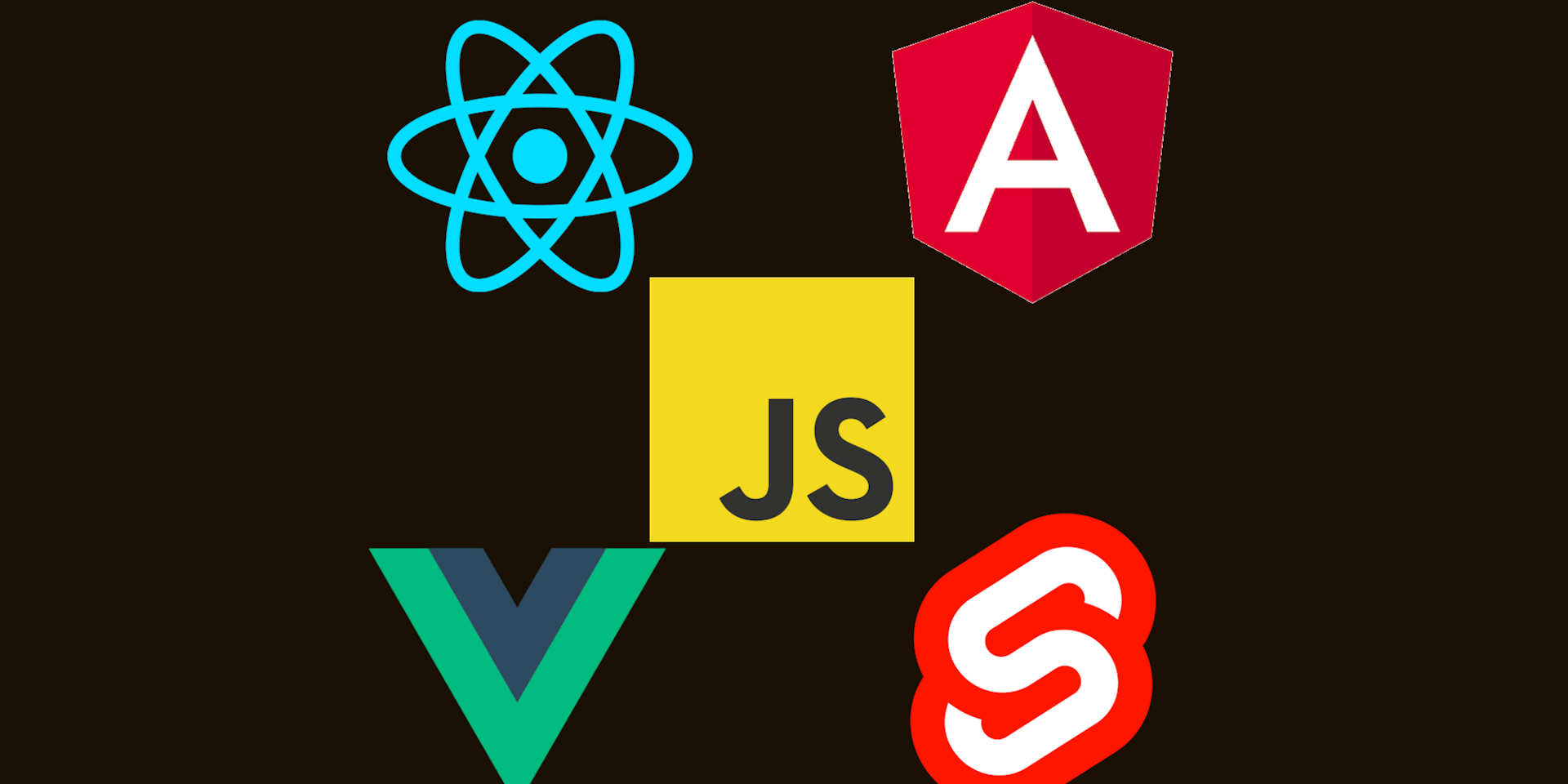
There are wayyyy too many Javascript frameworks. Simple thesis. Too many frameworks. There's React, Vue, Angular, Svelte, Vanilla, and those are just the ones I've used.
My first framework
Or should I say, none at all.
I started out vanilla JS, like any developer should (learning a framework without learning the fundamentals is setting yourself up for failure.) I loved every part of it... except... literally everything.
Vanilla, frameworkless, whatever you call it, is just the base javascript. And I'm talking basic basic, I didn't even know of the existence of typescript back in this dark age.
I only built a few apps using vanilla. This is partly because I realized frameworks are good, partly because I really only enjoyed web development when I started using a framework.
I was looking for a couple examples on my GitHub, but then I realized that most of my projects on GitHub use vanilla. This is for 2 reasons:
- I thought my code back then was actually good and functional, and that putting it on GitHub was a professional thing to do.
- I didn't know of other static hosting methods besides GitHub Pages. I now know that Pages is one of the least effective solutions, and should only really be used for simple documentation sites.
One simple example (my favorite) is Bundle. It is on GitHub and was my first actual project. It is a simple Minecraft bundle generator, I quickly coded it the day the bundle was released in a 1.17 snapshot.
I had seen many command generators like MCStacker which I had used, and wanted to make my own! Now thinking, this system could have been made like 1000x more efficient (I have a way to convert it from 68 lines to about 14). I refuse to update this though, because I want the nostalgia and record of the old goldies.
That system was a spaghetti coded mess of a machine, and only existed because I thought it was cool.
That was my first experience with Vanilla. I had one final project that I still develop to this day. It's been infamously named MinecraftPanel. I am proud of this machine because it works well.
I won't go into too much detail, because the existence is well documented with meaningful commit messages, and a good README. But in short, it is the last thing I made before moving to frameworks. It is built around JQuery and TypeScript. It allows you to initialize a Minecraft server from the terminal, then access a powerful web ui to manage it. I highly encourage you to mess around with it, because I literally use it every time I develop Minecraft plugins.
Aaaaand, the decision
Welp, it's time. I have acknowledged the existence of frameworks, and found the 3 most popular: React, Vue, and Angular.
Angular
Oh god, these guys. I have tried every single one of them, I have built at least some scale of app using React and Vue, and booted Angular right away.
If I recall correctly, Angular might have been the first one that I tried, but it just didn't click for me. Angular feels to "formal" if that's a way to say it. There is a strict way to write out each class, and it feels a lot more like a client side MVC than a framework.
Angular does work very reactively, and I have major respect for people who can use it well, I just can't. I hate rules, and Angular just has too many. The initial idea was good, but it crossed the line when I had to add an import to another file just to use their built in http module.
Vue
I really like Vue (I always pronounced it Voo
, only recently learned it's View
). Vue is a much less strict framework on how it works, and just, kind of, works...
Whereas Angular felt much more like a commitment to using it, Vue seemed like something I can just drop into an app. While that is an option, it wasn't the best for old me. I now very much prefer full application meta frameworks (Next.js, Nuxt.js, SvelteKit, etc.). Having to add Vue to an app is difficult, but Vue is powerful.
Vue is a good, and very reactive framework. It feels like a good compromise between full on HTMl in JS (JSX, React's approach), and JS in HTML (Angular's approach). I like how easy it is to create a loop (stole the idea for my framework), and the state system, just, makes sense!
My only real complaint with Vue is just that it didn't feel like it should work. The .vue files seemed to magical to old me, with the import's looking like they were in a different scope than the Components that I was importing. It worked though, and I will gladly make you an Vue app if that is what you ask for... Maybe
While I have developed like 2 full apps in Vue, neither are public just because I don't really think they are important enough for GitHub.
React (this took a while)
Finally (not finally), my current go-to framework.
I started learning React initially initially actually in June of 2021 (before anything else, that's right). But I don't count this.
React Native was what I was trying to learn. I just wanted to experiment with mobile development, and didn't want to learn Swift (I'm an apple user, deal with it).
I was ok with web tech at this point, but didn't really want to learn the React part of React Native...
So I gave up on it for the next 5 months until I started Wyvern.
Yep, my big project. My life's goal: Make a worse Discord
That is Wyvern, the Discord knock-off, and you know it is a knock-off.
Wyvern is my big project, I've taken breaks on it, but it is the big React project. I spent about a day learning React before starting it, thought I was ready, and fricked everything up.
This is something I will write about when I write about how I would have learned to code now that I know how to code (if I were to restart). For now, don't be dumb, don't start using a framework before learning it.
Anyways, let's rewind here. React is what the official Discord uses, and if I'm gonna copy it, I'm gonna copy everything (hehe). I actually really enjoy React, sept for those dumb bits with state.
I initially made Wyvern out of class components, then I had to rewrite the entire app because I learned that the modern React Router required functional components. If you are starting a React projects today, dont use class components. Use (not-so) functional components and Higher Order Components.
React has actually treated me quite well, and definitely makes writing the ui itself hella easy. I just missed the memo on useState with arrays.
React's useState hook is one of it's most used (just behind useEffect I think) because, well, it defines the state in your application. It is very simple:
function MyComponent() {
// you just write the value `name` and setter function `setName`, simple as pie right! (no)
const [name, setName] = useState("bob")
return (
<div>
<input onChange={(e) => setName(e.target.value)} />
<p>{name}</p>
</div>
)
}
It would be, but the way React handles arrays is quite unconventional. I don't want to go into great detail because I get physical pain just discussing it, but I recommend this article by Mohammed Nadeem Shareef that genuinely saved me from pulling out my microchip.
In the end for React, I won't be changing what Wyvern uses. If I could though, I would have chosen Svelte.
Quick end note: Next.js, which powers this website, is amazing. It has everything I ever wanted in a meta framework, and is why I will use it over Nuxt and SvelteKit (which is experimental, but quote me that it will become the best option eventually).
Svelte
I wanted to quickly acknowledge the existence of Svelte. Svelte is like everything I ever wanted in one. Initially, I thought it looked like Vue, but it is so much different. You just, declare variables! They become reactive!
Svelte is actually not a front end framework, but a compiler that compiles a .svelte files to vanilla javascript. It is much faster than any of the others, and will be what I use when I make a website that I will use to teach people how to code (coming soon, be on the lookout for a blog post about it!).
Svelte is like my favorite, I wanted to use Next.js for this, because I wasn't gonna make the Wyvern mistake of using a framework I've never used on a big project.
I have spent time learning Svelte, and will make some courses eventually on how to use it, and other frameworks!
The thesis
I said I was creating my own framework! Well duh, I'm not lying. It is gonna be called Reactive
(I'm so creative, I know).
I took inspiration from Vue and Alpine. This framework exists almost entirely in the dom, so it doesn't require any virtual dom like React, Vue, or Angular do.
The current framework is 1Kb. It is subject to change, because I am still developing it, but I love the way it works.
This framework was not designed to be the fastest thing in the world, far from it. It kinda sucks at everything, I just want it simple.
I want anyone to be able to take this single script file, throw it into a completely static site, and just add a few bits of interactivity to it.
Thinking about it, it also works as a fullstack framework, although I hiiiiiiighly (I need more i's but I don't want to) discourage it.
The values of every variable is stored initially in the dom, not javascript. Once the framework loads, the variables are loaded into memory, but initially, they are just dom. This means you can assign to them in the server render, then have the client pick up exactly where the server left off.
Again, I don't think anyone would ever use it as a server framework, I see it more for simple things. Like the below comments system, would be great to make Reactive
Alright, I'm gonna stop before I run out of memory. This was a journey, and this post took a very long time to write. See you next time, cheers!
Related Posts
Why I chose to rewrite TKDKid1000's Website with Firebase and Next.js
The 2 older versions of TKDKid1000's website worked, but they had their issues. In this article, I explain the history of these websites, and why and how I'm switching to Next.js and Firebase.
You must be logged in to comment!